ImageEn, unit iexHelperFunctions |
|
TIEBitmapHelper.OCR_GetRegions
Declaration
function OCR_GetRegions(out Regions: TIERectArray; out TextResults: TIEStringArray; GetText: Boolean; const LanguageCode: string; SegmentationMode: TIEVisionOCRPageSegmentationMode = ievOCRAuto; const LanguagePath: string = ''; Engine: TIEVisionOCREngine = ievOCRDefault): Integer; overload;
function OCR_GetRegions(out Regions: TIERectArray; out TextResults: TIEStringArray; GetText: Boolean; Language: TIEOCRLanguages = OCR_English_Language; SegmentationMode: TIEVisionOCRPageSegmentationMode = ievOCRAuto; const LanguagePath: string = ''; Engine: TIEVisionOCREngine = ievOCRDefault): Integer; overload;
Description
A shortcut method that creates a
TIEVisionOCR object and calls
getRegions to return all text regions in the image (Filling the Regions parameter).
If GetText is enabled, it will also perform
OCR on each region and fill the TextResults parameter. Set to false if you only need regions as it will be much faster.
The language can be specified by
type or as a language code string (e.g. 'eng').
Optionally you can specify the
segmentation mode, path that contains the language file and
OCR engine.
Result is the number of found regions (count of Regions and TextResults arrays).
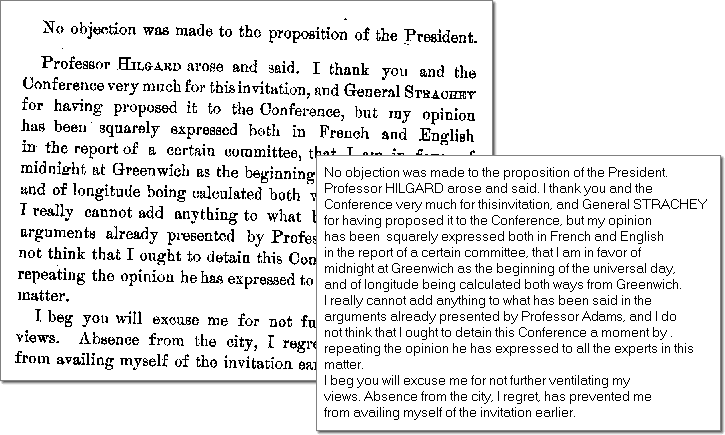
Note:
◼You must add the iexHelperFunctions unit to your uses clause
◼LanguagePath and Engine cannot be changed after first use (without restarting the application)
◼You wil require a TrainedData file for the specified language (e.g. "eng.TrainedData"). An exception is raised if it is not found
◼OCR requires
IEVision. You will need to
register it before calling the method
◼If the bitmap is attached to a
TImageEnView or
TImageEnIO, then
OnProgress and
OnFinishWork will occur during processing
Method Behaviour
The following call:
ImageEnView1.IEBitmap.OCR_GetRegions( outRects, outTexts, True, OCR_English_Language, ievOCRAuto );
for i := 0 to Length(outRects)-1 do
begin
r := outRects[i];
Memo1.Lines.Add( format( '%d,%d,%d,%d: %s', [ r.x, r.y, r.x + r.width, r.y + r.height, TextResults[i] ]);
end;
Is the same as calling:
// Create OCR Engine
m_OCR := IEVisionLib.createOCR( PAnsiChar( AnsiString( langPath )), PAnsiChar( AnsiString( langCode )), ievOCRDefault );
m_OCR.setSegmentationMode( ievOCRAuto );
// detect regions and perform OCR
regions := m_OCR.getRegions( ImageEnView1.IEBitmap.GetIEVisionImage(), Skip_Image_Regions );
for i := 0 to regions.size()-1 do
begin
r := regions.getRect(i);
str := String( m_OCR.recognize( ImageEnView1.IEBitmap.GetIEVisionImage(), r ).c_str() );
Memo1.Lines.Add( format( '%d,%d,%d,%d: %s', [ r.x, r.y, r.x + r.width, r.y + r.height, str ]);
end;
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Find all the text regions of document image in ImageEnView1 and output them as text layers in ImageEnView2
// Note: OnProgress and OnFinishWork will occur for ImageEnView1
procedure TMainForm.btnGetRegionsClick(Sender: TObject);
const
Color_List : array [0..12] of TColor = (clAqua, clBlue, clFuchsia, clGray, clGreen, clLime,
clMaroon, clNavy, clOlive, clPurple, clRed, clTeal, clYellow);
var
angle: single;
i: integer;
r: TRect;
outRects: TIERectArray;
outTexts: TIEStringArray;
begin
ShowTempHourglass();
// Auto-rotate document
angle := ImageEnView1.IEBitmap.OCR_GetOrientation();
if angle <> 0 then
ImageEnView1.Proc.Rotate( -angle, ierFast, clWhite );
// Create a blank image the same size as the original image to show our text
ImageEnView2.IEBitmap.Allocate( ImageEnView1.IEBitmap.Width, ImageEnView1.IEBitmap.Height, clWhite );
ImageEnView2.Fit();
ImageEnView2.LayersClear( False );
// Get our region and text
ImageEnView1.IEBitmap.OCR_GetRegions( outRects, outTexts, True, OCR_English_Language );
for i := 0 to Length(outRects)-1 do
begin
r := outRects[i];
// Mark this region in the source image (create a semitransparent layer over the rectangle)
ImageEnView1.LayersAdd( r.width, r.height, ie24RGB, r.Left, r.Top );
ImageEnView1.CurrentLayer.Bitmap.Fill( Color_List[i mod length(Color_List)] );
ImageEnView1.CurrentLayer.Transparency := 80;
// Draw each text region to ImageEnView2
ImageEnView2.LayersAdd( ielkText, r );
With TIETextLayer( ImageEnView2.CurrentLayer ) do
begin
Text := outTexts[i];
Font.Name := 'Tahoma';
Font.Height := trunc( sqrt( width * height * 2 / Length( outTexts[i] ))); // Font size may be wrong, this is just an example!!
Font.Color := clBlack;
BorderColor := clNone;
FillColor := Color_List[i mod length(Color_List)];
end;
end;
ImageEnView1.Update();
ImageEnView2.Update();
end;
See Also
◼TIEOCRLanguages◼LanguageExistsInFolder◼IEVisionLanguageCodeToName◼IEVisionLanguageNameToCode