Properties · Methods · Events · Demos · Examples
Declaration
TImageEnIO = class(TComponent);
Description
TImageEnIO provides input/output support to ImageEn:
◼Loading and
saving images
◼Access to
image properties and meta-data
◼Acquisition of images from cameras and scanners
◼Printing
◼DirectShow functions for multimedia support
See also:
TImageEnMIO, which works with multiple frame images (e.g. animated GIFs)
Generally you will not add a TImageEnIO component directly to your project. It is accessed via the following methods:
ImageEnView1.IO.LoadFromFile('C:\MyImage.jpeg');
ImageEnView1.IO.DoPrintPreviewDialog();
2. Using the IO helper property of a TIEBitmap, TBitmap or TImage
// Scan an image and save to file
bmp := TIEBitmap.Create();
if bmp.IO.Acquire() then
bmp.IO.SaveToFile( 'D:\scan.pdf' );
bmp.Free;
3. Attached to a TIEBitmap or TBitmap in code
// Scan an image and save to file
bmp := TBitmap.Create();
io := TImageEnIO.CreateFromBitmap(bmp);
if io.Acquire() then
io.SaveToFile( 'D:\scan.pdf' );
io.Free();
bmp.Free();
4. Attached to a TImage
Note: In this case a TImageEnIO would be added to the form and the
AttachedTImage set appropriately.
Notes
◼Ensure you do not call any TImageEnIO methods before it is actually attached to an image container (
TImageEnView,
TIEBitmap, etc)
◼For the
TImageEnMView component use a
TImageEnMIO rather than a TImageEnIO
Demo | Description | Demo Project Folder | Compiled Demo |
Annotations in Meta-Data | Add text, lines and highlighting to an image and store them in meta-data | InputOutput\Annotations\Annotations.dpr | |
Camera Raw Files | Loading digital camera raw files using the ielib.dll plug-in | InputOutput\CameraRaw\CameraRaw.dpr | |
Dicom Viewer | Read and animate images of a Dicom file | InputOutput\Dicom\Dicom.dpr | |
EXIF Editor | View and edit EXIF fields from a digital camera files (JPEG, RAW, etc) | InputOutput\EXIF\EXIF.dpr | |
Image Loading Performance | Options to improve loading performance with TImageEnView | InputOutput\IEViewPerformance\Performance.dpr | |
IPTC Editor | View and edit IPTC fields from a JPEG or TIFF image | InputOutput\IPTC\IPTC.dpr | |
Geo Maps | Use the GPS data in photos to show their location on a map | InputOutput\GeoMaps\GeoMaps.dpr | |
Multi-Page Image Loading | Using ImageEnView buttons to navigate the images stored in a multiple-frame file, such as TIFF, GIF, MPEG, PDF, etc. | InputOutput\IEViewMulti\IEViewMulti.dpr | |
PDF Builder | Create PDF and PS files from a selection of images | PDF\PDFBuilder\PDFBuilder.dpr | |
Preload Images | Loading images in the background and displaying as required | InputOutput\Preload\Preload.dpr | |
Print Selection | Print only the selected area of an image | InputOutput\PrintSelected\PrintSelected.dpr | |
Printing Demo | Printing and print preview demo | InputOutput\PrintProjects\PrintProjects.dpr | |
True Raw Bitmap Format | Loading and saving images in a true "Raw" format (Note: this is not the same as camera raw format) | InputOutput\RealRAW\RealRAW.dpr | |
XMP Meta Data | Display common XMP fields from JPEG, TIFF and PSD files | InputOutput\XMP\XMP.dpr | |
Acquire from Any Source | Acquisition from any source: Twain, WIA and connected cameras and cards | ImageAcquisition\AllAcquire\AllAcquire.dpr | |
All Actions | Demo showing all ImageEn actions (400+) available to rapidly build applications | Actions\AllActions\AllActions.dpr | |
// Convert a PNG to JPEG at 90% quality
ImageEnView1.IO.LoadFromFile('D:\image.png');
ImageEnView1.IO.Params.JPEG_Quality := 90;
ImageEnView1.IO.SaveToFile('D:\output.jpg');
// Save a TIFF with CMYK
ImageEnView1.IO.LoadFromFile('D:\image.tif');
ImageEnView1.IO.Params.TIFF_PhotometInterpret := ioTIFF_CMYK;
ImageEnView1.IO.SaveToFile('D:\image-cmyk.tif');
// Scan an image at 300dpi
ImageEnView1.IO.AcquireParams.YResolution := 300;
ImageEnView1.IO.AcquireParams.XResolution := 300;
ImageEnView1.IO.Acquire();
// Enable duplexing on the current scanner
ImageEnView1.IO.AcquireParams.DuplexEnabled := True;
// Show advanced GIF saving properties
ImageEnView1.IO.SimplifiedParamsDialogs := False;
ImageEnView1.IO.DoPreviews([ppGIF]);
// Prompt to print the current image
ImageEnView1.IO.DoPrintPreviewDialog( iedtDialog );
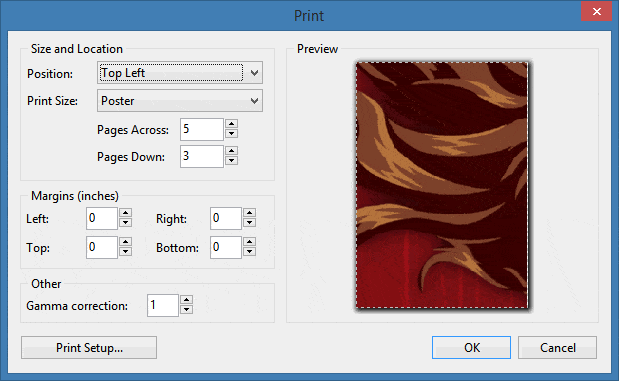
Connected Component
Generic Input/Output
Image Acquisition (Twain/WIA)
Aynchronous Input/Output
Video Capture
Dialogs
Printing
Format-Specific Methods
Adobe PDF
Adobe PSD
AVI Videos
BMP
BmpRaw (a true "raw" bitmap format, not a camera Raw file)
Camera Raw Files
Cursors (CUR)
DICOM Medical Imaging Format
GIF
Icons (ICO)
IEN (ImageEn native image format with layers)
JPEG
JPEG 2000
Media Files (AVI, MPEG, WMV..)
Meta Files (WMF, EMF)
Microsoft HD Photos/JPEG XR (WDP/JXR/HDP)
PCX and DCX (Multipage PCX)
PNG
PostScript (PS)
PXM (PPM, PBM, PGM)
SVG (Scalable Vector Graphics)
Targa (TGA)
TIFF
WebP
WBMP
WIC Plug-In Formats (such as HEIF/HEIC, DirectDraw Surface, etc)
ZIP
Loading contents...