TIEPolylineLayer.EnableComplexPath
Declaration
property EnableComplexPath: Boolean;
Description
Enables path points to support
additional properties such as actions, LineTo, MoveTo, etc, as well as bezier and quadratic curves, and arcs.
You do not need to enable
EnableComplexPath, as it is enabled automatically in the following situations:
◼If you
set points with the
TIEPointFormat overload
◼If you specify as
SVG path tag◼If you
import an SVG file that contains a path tag
If you set
EnableComplexPath to false, the point formatting will be cleared which may change how the layer looks.
Default: False
Comparing Complex Methods 1
Three alternative methods to create a complex polyline layer:
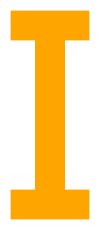
// Set path using SVG tag
ImageEnView1.LayersAdd( ielkPolyline );
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.AsSVG := '<path d="M 10,10 L 90,10 M 50,10 L 50,190 M 10,190 L 90,190" stroke="orange" stroke-width="30" fill="none" />';
ImageEnView1.Update();
// Set path using arrays of TDPoint and TIEPointFormat
ImageEnView1.LayersAdd( ielkPolyline );
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.BorderColor := clWebOrange;
polyLayer.BorderWidth := 30;
polyLayer.FillColor := clNone;
polyLayer.PolylineClosed := False;
SetLength( pts, 6 );
SetLength( fmts, 6 );
fmts[0].Action := iepaMoveTo;
pts[0] := DPoint( 10, 10 );
fmts[1].Action := iepaLineTo;
pts[1] := DPoint( 90, 10 );
fmts[2].Action := iepaMoveTo;
pts[2] := DPoint( 50, 10 );
fmts[3].Action := iepaLineTo;
pts[3] := DPoint( 50, 190 );
fmts[4].Action := iepaMoveTo;
pts[4] := DPoint( 10, 190 );
fmts[5].Action := iepaLineTo;
pts[5] := DPoint( 90, 190 );
polyLayer.SetPoints( pts, fmts, iepbBitmap );
ImageEnView1.Update();
// Set path using AddPath overload
ImageEnView1.LayersAdd( ielkPolyline );
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.BorderColor := clWebOrange;
polyLayer.BorderWidth := 30;
polyLayer.FillColor := clNone;
polyLayer.PolylineClosed := False;
polyLayer.AddPoint( 10, 10, iepbBitmap, iepaMoveTo );
polyLayer.AddPoint( 90, 10, iepbBitmap, iepaLineTo );
polyLayer.AddPoint( 50, 10, iepbBitmap, iepaMoveTo );
polyLayer.AddPoint( 50, 190, iepbBitmap, iepaLineTo );
polyLayer.AddPoint( 10, 190, iepbBitmap, iepaMoveTo );
polyLayer.AddPoint( 90, 190, iepbBitmap, iepaLineTo );
ImageEnView1.Update();
Comparing Complex Methods 2
Three alternative methods to create a complex curved polyline layer:
// Set path using SVG tag
ImageEnView1.LayersAdd( ielkPolyline );
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.AsSVG := '<path d="M 70,30 C 130,-30 130,90 70,30 C 10,90 10,-30 70,30 Z" style="fill:yellow; stroke:green; stroke-width:3" />';
ImageEnView1.Update();
// Set path using arrays of TDPoint and TIEPointFormat
ImageEnView1.LayersAdd( ielkPolyline );
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.BorderColor := clGreen;
polyLayer.BorderWidth := 3;
polyLayer.FillColor := clYellow;
SetLength( pts, 3 );
SetLength( fmts, 3 );
// M 70,30
fmts[0].Action := iepaMoveTo;
pts[0] := DPoint( 70, 30 );
// C 130,-30 130,90 70,30
fmts[1].Action := iepaBezierCurveTo;
fmts[1].CurveX1 := 130;
fmts[1].CurveY1 := -30;
fmts[1].CurveX2 := 130;
fmts[1].CurveY2 := 90;
pts[1] := DPoint( 70, 30 );
// C 10,90 10,-30 70,30 Z
fmts[2].Action := iepaBezierCurveTo;
fmts[2].CurveX1 := 10;
fmts[2].CurveY1 := 90;
fmts[2].CurveX2 := 10;
fmts[2].CurveY2 := -30;
pts[2] := DPoint( 70, 30 );
fmts[2].ClosePath := True;
polyLayer.SetPoints( pts, fmts, iepbBitmap );
ImageEnView1.Update();
// Set path using AddPath overload
ImageEnView1.LayersAdd( ielkPolyline );
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.BorderColor := clGreen;
polyLayer.BorderWidth := 3;
polyLayer.FillColor := clYellow;
// M 70,30
polyLayer.AddPoint( 70, 30, iepbBitmap, iepaMoveTo );
// C 130,-30 130,90 70,30
polyLayer.AddPoint( 70, 30, iepbBitmap, iepaBezierCurveTo, False, 130, -30, 130, 90 );
// C 10,90 10,-30 70,30 Z
polyLayer.AddPoint( 70, 30, iepbBitmap, iepaBezierCurveTo, True, 10, 90, 10, -30 );
ImageEnView1.Update();
// Add the following SVG Arc path: <path fill="#0c64d2" d="M49 58 A42 42 10 1 0 50 58z"/>
ImageEnView1.LayersAdd( ielkPolyline );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 49, 58, iepbBitmap, iepaMoveTo );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).AddPoint( 50 58, iepbBitmap, iepaArcTo, True, 42, 42, 10, True, False );
ImageEnView1.Update();