Declaration
// Extract tags overload
function ParseSVG(const SVGContent: String; var DestTags: TIESVGTags; var TagCount: Integer; ExtractTSpans: Boolean = True): Boolean; overload;
function ParseSVG(const FileName: String; Stream: TStream; var DestTags: TIESVGTags; var TagCount: Integer; ExtractTSpans: Boolean = True): Boolean; overload;
// Rendering overload
function ParseSVG(const SVGContent: string; DestBitmap: TIEBitmap; ImportWidth: Integer = -1; ImportHeight: Integer = -1; MaintainAR: Boolean = False): Boolean; overload;
function ParseSVG(const FileName: WideString; Stream: TStream; DestBitmap: TIEBitmap; ImportWidth: Integer = -1; ImportHeight: Integer = -1; MaintainAR: Boolean = False; ParamsOnly: Boolean = False): Boolean; overload;
// Layer importing overload
function ParseSVG(const SVGContent: string; DestImageEnView: TIEView; Append: Boolean; ImproveLayout: Boolean = True): Integer; overload;
function ParseSVG(const FileName: String; Stream: TStream; DestImageEnView: TIEView; Append: Boolean; ImproveLayout: Boolean = True): Integer; overload;
Description
Parse an SVG file or stream.
Tags can be output as an array, imported as layers into a
TImageEnView (though you are better to use
LayersImport) or rendered to a
TIEBitmap (though you are better to use
ImportFromFileSVG).
If ExtractTSpans = True, any embedded TSpans will be converted to text tags.
For the TIEBitmap overload, set
ImportWidth and
ImportHeight to the desired image size. If -1 is specified for both, the original image size is used.
To maintain the image aspect ratio set only one size, assigning -1 to the other, e.g. ImportFromFileSVG( 'axi.svg', -1, 500 ); or set both values and enable
MaintainAR.
Result is false if the tag could not be interpreted.
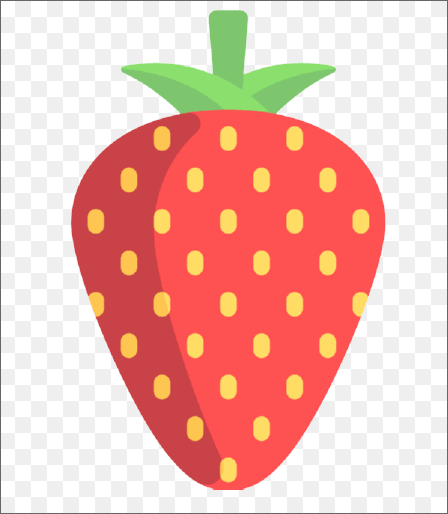
Demo
| Demos\InputOutput\SVGParsing\SVGParsing.dpr |
Examples
// Add a description of all SVG tags to a TMemo
lbxSvgTags.Items.BeginUpdate();
svgParser := TIESVGParser.Create();
try
svgParser.ParseSVG( Filename, nil, svgTags, svgTagCount );
for i := 0 to Length( svgTags ) - 1 do
begin
s := svgTags[i].Content;
if Length( s ) > MAX_LENGTH then
begin
SetLength( s, MAX_LENGTH );
s := s + '...';
end;
lbxSvgTags.Lines.Add( s );
end;
finally
lbxSvgTags.Items.EndUpdate();
svgParser.Free();
end;
// Manually render an SVG
svgParser := TIESVGParser.Create();
try
// Note: This will be the same as ImageEnView3.IO.ImportFromFileSVG() if Skia or ImageMagick is not being used
svgParser.ParseSVG( mySvgContent, ImageEnView1.IEBitmap, -1, -1, True );
ImageEnView1.Fit( False );
ImageEnView1.Update();
finally
svgParser.Free();
end;
See Also
◼ IEParseSVGImageTag◼ IEParseSVGLineTag◼ IEParseSVGPathTag◼ IEParseSVGPolyTag◼ IEParseSVGShapeTag◼ IEParseSVGTextTag◼ SVG Implementation Status