TIEVisionImage.thinPlateSplineShapeTransform
TIEVisionImage.thinPlateSplineShapeTransform
Declaration
function thinPlateSplineShapeTransform(srcPoints: TIEVisionVectorPoint2f; dstPoints: TIEVisionVectorPoint2f; regularization: double = 25000.0): TIEVisionImage; overload; safecall;
function thinPlateSplineShapeTransform(srcPoints: TIEVisionVectorPoint2f; dstPoints: TIEVisionVectorPoint2f; borderType: TIEVisionBorderType; borderValue: TIEVisionScalar; regularization: double = 25000.0): TIEVisionImage; overload; safecall;
Description
Apply a Thin Plate Spline Shape Transformation to an image.
srcPoints and dstPoints specifying the starting and ending position of each tranformation point, e.g. srcPoints[0] specifies the original position of the first point, and dstPoints[0] specifies the position to shift it to.
The regularization parameter relaxes the exact interpolation requirements of the TPS algorithm.
borderType specifies what to fill in newly created border areas. Generally this will be ievBORDER_REPLICATE which replicates existing content, or ievBORDER_CONSTANT which uses the specified RGB border color (see example below).
Returns the warped image.
Note:
◼This method is available as an
interactive tool by setting ImageEnView1.MouseInteractGeneral := [miTransformTool];
◼Also consider
Warp which performs point based warping
| IEVision\TPSShapeTransform\TPSShapeTransform.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
Border Fill Comparison
// Original image
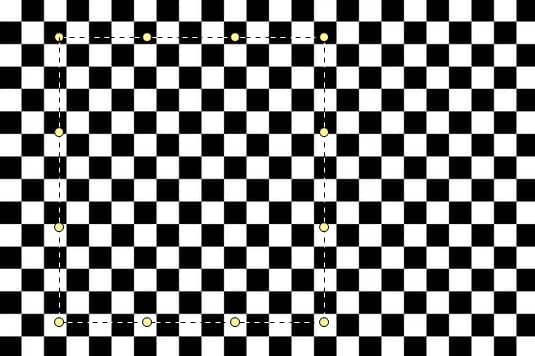
// Fill new borders areas with gray
rgb := TColor2TRGB( clGray );
img := ImageEnView1.IEBitmap.GetIEVisionImage().thinPlateSplineShapeTransform( srcPoints, dstPoints, ievBORDER_CONSTANT, IEVisionScalar( rgb.b, rgb.g, rgb.r ));
ImageEnView1.IEBitmap.AssignIEVisionImage( img );
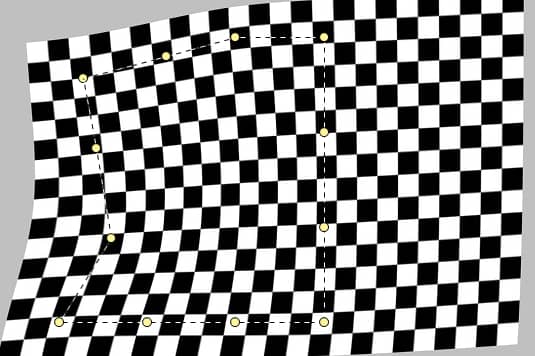
// Replicate existing content when filling new border areas
img := ImageEnView1.IEBitmap.GetIEVisionImage().thinPlateSplineShapeTransform( srcPoints, dstPoints, ievBORDER_REPLICATE, IEVisionScalar( 0,0,0 ));
ImageEnView1.IEBitmap.AssignIEVisionImage( img );
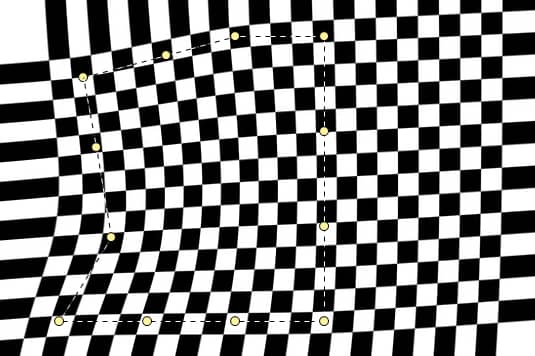
// Apply a Thin Plate Spline Shape Transformation to an image
// Load test image
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
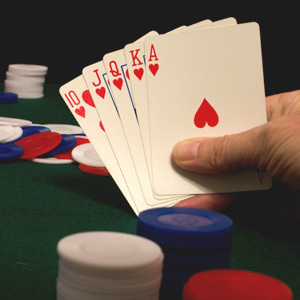
srcPoints := IEVisionLib().createVectorPoint2f();
dstPoints := IEVisionLib().createVectorPoint2f();
// Create a four point selection of the middle third of the image
// TL
srcPoints.push_back( IEVisionPoint2f( 50, 50 ));
dstPoints.push_back( IEVisionPoint2f( 50, 50 ));
// TR
srcPoints.push_back( IEVisionPoint2f( 250, 50 ));
dstPoints.push_back( IEVisionPoint2f( 250, 50 ));
// BR
srcPoints.push_back( IEVisionPoint2f( 50, 250 ));
dstPoints.push_back( IEVisionPoint2f( 50, 250 ));
// BL
srcPoints.push_back( IEVisionPoint2f( 250, 250 ));
dstPoints.push_back( IEVisionPoint2f( 300, 300 )); // Offset bottom right to edge
im := SourceIEViewer.IEBitmap.GetIEVisionImage().thinPlateSplineShapeTransform( srcPoints, dstPoints );
DestIEViewer.IEBitmap.AssignIEVisionImage( im );
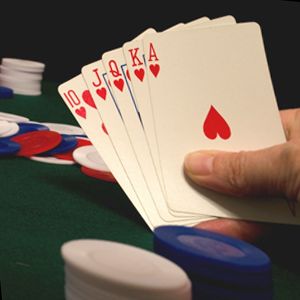
srcPoints := IEVisionLib().createVectorPoint2f();
dstPoints := IEVisionLib().createVectorPoint2f();
for i := 0 to 11 do // 12 points in our transformation array
begin
srcPoints.push_back( IEVisionPoint2f( SrcPts[i].X, SrcPts[i].Y ));
dstPoints.push_back( IEVisionPoint2f( DstPts[i].X, DstPts[i].Y ));
end;
img := ImageEnView1.IEBitmap.GetIEVisionImage().thinPlateSplineShapeTransform(srcPoints, dstPoints);
ImageEnView1.IEBitmap.AssignIEVisionImage(img);
See Also
◼TIETransformToolInteraction
◼MouseInteractGeneral