Properties · Methods · Demos · Examples
Declaration
TIEPdfViewer = class(TIEUserInteraction);
Description
A class of
TIEUserInteraction that allows viewing, editing and saving PDF files. It is embedded in
TImageEnView as
PdfViewer.
Features
* Custom ImageEn features (not available in standard PDFium)
Note:
◼PDFium support requires Delphi/BCB 7 or newer
◼The
PDFium plug-in DLL must exist in the same folder as your application EXE. It is included in the ImageEn installation folder under \DLL\PDF\
◼Read more about
ImageEn PDF Support
◼You cannot use the
PdfViewer with multiple
layers
◼The size of the PDF page is displayed on-screen and when
output to bitmap is controlled by
IEGlobalSettings().PdfViewerDefaults.DPI
Mouse Interactions
Typically the following
mouse interactions are used with PdfViewer:
Interactions | Description |
[ miPdfSelectText, miPdfSelectRgn ] | The user can select text or a region (as an image) based on what is under the cursor |
[ miPdfSelectText ] | The user can select text |
[ miPdfSelectRgn ] | The user can select a rectangular region (which can be copied to the clipboard as an image) |
[ miScroll, miZoom ] | The user can click and drag to scroll the page or left/right click to zoom in/out |
[ miPdfSelectObject ] | The user can select and edit objects of the PDF page |
[ miPdfSelectAnnotation ] | The user can select and edit annotations of the PDF page |
When enabling PdfViewer,
MouseInteractGeneral will be set to [miPdfSelectText, miPdfSelectRgn]. You can edit objects using miPdfSelectObject, and annotations using miPdfSelectAnnotation. miPdfSelectRgn, miPdfSelectObject and miPdfSelectAnnotation have no effect if
ShowAllPages is enabled.
The following
interactions are supported: miPdfSelectText, miScroll, miZoom, miSmoothZoom, miDblClickZoom, miMovingScroll. If not
showing all pages, you can also use: miPdfSelectRgn, miSelectZoom, miSelect, miSelectPolygon, miSelectCircle, miSelectMagicWand, miSelectLasso, miSelectChromaKey
All
layer interactions are disabled.
You can also use the mouse to edit form fields if you enable
AllowFormEditing.
Keyboard Shortcuts
Shortcut | Description |
Ctrl+C/X/V | Cut/Copy/Paste within a form field |
Ctrl+C | Copy selected text or region/image |
Ctrl+A | Select all text on page or in current form field |
PageUp/Down | Go to previous/next page |
Home/End | Go to first/last page |
Cursor keys | Scroll the image (requires iesoAllowMoveByKeyboard) |
Page Editing
Users can edit objects in a PDF page if you set
MouseInteractGeneral to [miPdfSelectObject] and enable
AllowObjectEditing, and edit annotations by setting
MouseInteractGeneral to [miPdfSelectAnnotation] and enabling
AllowAnnotationEditing.
When editing is enabled:
◼Objects and
annotations can be selected
◼Selected objects can be size and moved (by mouse or cursor keys)
◼Pressing Delete will remove the current object
◼Clipboard operations are supported, including pasting text or images from the clipboard (objects only, not annotations)
| Demos\PDF\PDFViewer\PdfViewer.dpr |
| Demos\Actions\Actions_PdfViewer\PdfViewerActions.dpr |
| Demos\PDF\PDFViewerToolbar\PdfViewerToolbar.dpr |
| Demos\PDF\PDFAnnotations\PdfAnnotations.dpr |
| Demos\PDF\PDFPageObjects\PDFPageObjects.dpr |
| Demos\PDF\PDFPageDragDrop\PDFPageDD.dpr |
| Demos\PDF\PDFViewerFormFields\PdfViewerFF.dpr |
| Demos\PDF\PDFPrinter\PdfPrinter.dpr |
// Register the PDFium Plug-In
IEGlobalSettings().RegisterPlugIns([ iepiPDFium ]);
// Display a PDF document (and allow text and image selection, scaled viewing, etc)
ImageEnView1.PdfViewer.Enabled := True;
ImageEnView1.MouseInteractGeneral := [ miPdfSelectText, miPdfSelectRgn ];
ImageEnView1.IO.LoadFromFilePDF( 'C:\document.pdf' );
// Show all pages at once
ImageEnView1.PdfViewer.ShowAllPages := True;
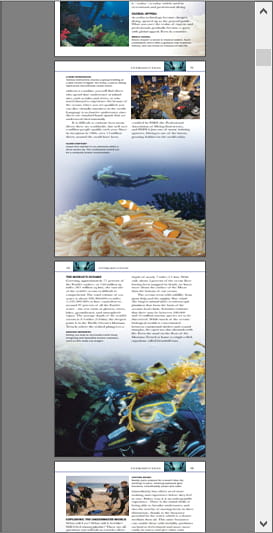
// Enable form editing
ImageEnView1.PdfViewer.Enabled := True;
ImageEnView1.PdfViewer.AllowFormEditing := True;
ImageEnView1.IO.LoadFromFilePDF( 'C:\document.pdf' );
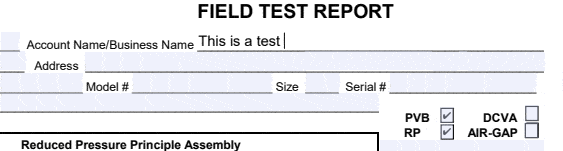
// Allow user to select images or text and copy to clipboard (automatically detecting selection based on what is under the cursor)
ImageEnView1.MouseInteractGeneral := [ miPdfSelectText, miPdfSelectRgn ];
ImageEnView1.PdfViewer.Enabled := True;
// Allow user to select text and copy to clipboard (image selection disabled)
ImageEnView1.MouseInteractGeneral := [ miPdfSelectText ];
ImageEnView1.PdfViewer.Enabled := True;
// Allow user to select images and copy to clipboard (text selection disabled)
ImageEnView1.MouseInteractGeneral := [ miPdfSelectRgn ];
ImageEnView1.PdfViewer.Enabled := True;
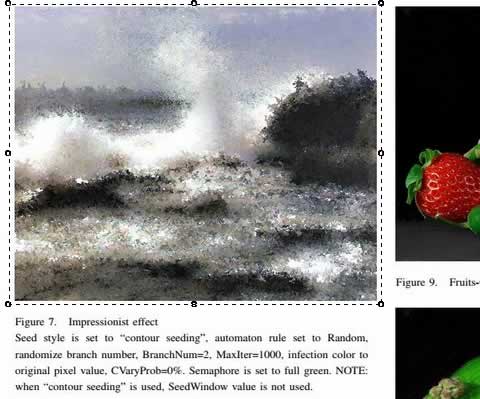
// Display a PDF document and allow editing of the page objects and annotations
ImageEnView1.PdfViewer.Enabled := True;
ImageEnView1.MouseInteractGeneral := [ miPdfSelectObject, miPdfSelectAnnotation ];
ImageEnView1.PdfViewer.AllowObjectEditing := True;
ImageEnView1.IO.LoadFromFilePDF( 'C:\document.pdf' );
// Merge two PDF documents
ImageEnView1.PdfViewer.Enabled := True;
ImageEnView1.IO.LoadFromFilePDF( 'C:\document.pdf' );
ImageEnView1.PdfViewer.ImportPages( 'C:\morepages.pdf' );
ImageEnView1.IO.SaveToFilePDF( 'C:\merged.pdf' );
// Move pages to the start of the document
ImageEnView1.PdfViewer.MovePages( [3, 4, 8, 9], 0);
// Delete pages from the document
ImageEnView1.PdfViewer.DeletePages([ 5, 6 ]);
// Prompt user to save changes
if ImageEnView1.PdfViewer.DocModified then
if MessageDlg( 'Save doc changes?', mtConfirmation, [ mbYes,mbNo ], 0 ) = mrYes then
ImageEnView1.IO.SaveToFilePDF( ImageEnView1.IO.Params.Filename );
// Rotate document right for display
ImageEnView1.PdfViewer.ViewRotation := iepr90Clockwise;
// Rotate current page only (updating the document)
ImageEnView1.PdfViewer.PageRotation[ ImageEnView1.PdfViewer.PageIndex ] := iepr90Clockwise;
// Find and select the text "Adobe"
ImageEnView1.PdfViewer.Find( 'Adobe' );
// Highlight the text "Adobe" thoughout the document
ImageEnView1.PdfViewer.HighlightText( 'Adobe' );
// Copy all text in the page to the clipboard
ImageEnView1.PdfViewer.SelectAll();
ImageEnView1.PdfViewer.CopyToClipboard();
// Save all text in the page to a file
ss := TStringList.Create;
ImageEnView1.LockPaint();
ImageEnView1.PdfViewer.SelectAll();
ss.Text := ImageEnView1.PdfViewer.SelText;
ImageEnView1.PdfViewer.ClearSelection();
ImageEnView1.UnlockPaint();
ss.SaveToFile( 'D:\Page.txt' );
ss.Free;
// TIEPdfViewer can also be used non-visually
pdf1 := TIEPdfViewer.Create();
pdf2 := TIEPdfViewer.Create();
pdf1.LoadFromFile( 'D:\Doc1.pdf' );
pdf2.LoadFromFile( 'D:\Doc2.pdf' );
pdf1.ImportPages( pdf2.Document );
pdf1.SaveToFile( 'D:\MergedDoc.pdf' );
pdf1.Free;
pdf2.Free;
// Show thumbnail preview of all pages of a PDF document
ImageEnView1.PdfViewer.Enabled := True;
ImageEnMView1.AttachedImageEnView := ImageEnView1;
ImageEnView1.IO.LoadFromFilePDF( 'C:\document.pdf' );
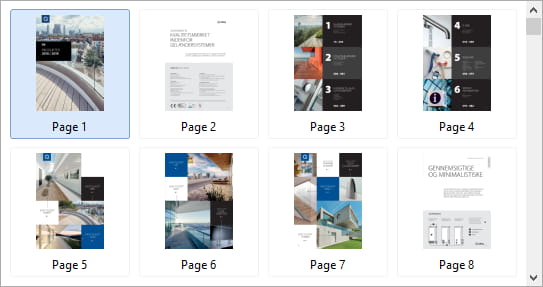
Display Properties
Document Properties
Document Page Editing
Clipboard Methods
Text Selection
Form Field Editing
Object Editing
Search and Highlight
Attachments
Other
See Also
◼PDFium PlugIn
◼PdfViewer
◼LoadFromFilePDF
◼SaveToFilePDF
◼PdfViewerDefaults